Hey folks! In this blog post, I will cover how we can integrate two salesforce orgs.
Nowadays we have many requirements where we integrate 3rd party Apps with the Salesforce Platform. Being a Salesforce developer I have also completed many integration projects. (For example Salesforce with Shopify, Salesforce with USAePay, Custom APIs). The integration can be a data sync and process sync. I personally like working on integration projects because every integration project is different from the previous one.
Sometimes a client can have multiple Salesforce orgs for as per their requirements. I recently have worked on a project where I have integrated one Salesforce org to another Salesforce org. In this project, I had a custom Apex REST resource in a Salesforce org (for reference we will call this new Salesforce org ). The client wants to call this Apex REST resource from another Salesforce Org (For reference we will call this old Salesforce org).
For this project, I have used Named Credentials for authenticating the API callouts. Named Credentials are really useful for authenticating API callouts from Salesforce to 3rd party apps. Salesforce manages all authentication for Apex callouts that specify a named credential as the callout endpoint so that your code doesn’t have to. In this project, We set up an API user with appropriate permissions in New Salesforce Org. We use this API user for this integration project. This is a best practice to use an API user for an integration project.
Here are the following steps I used to integrate with another Salesforce org.
Create an Apex REST resource (New Salesforce org)
First, we create an Apex REST API in the new sandbox. We will call this custom REST API from the Old Salesforce Org.
Following is a Sample apex REST class that can be used to get the account name by using a valid salesforce Id. This API can be called from any 3rd party platform.
Sample Apex Code
@RestResource(urlMapping='/SampleRestAPI/*')
global with sharing class SampleRestAPIRestController {
@HttpPost
global static void doPost(String SfId) {
RestResponse res = RestContext.response ;
Savepoint sp = Database.setSavepoint();
try {
//validations
if (String.isBlank(SfId)) {
res.responseBody = Blob.valueOf(JSON.serialize(new errorMessageWrapperClass(‘Missing Required fields’, ‘SfId is required!!’)));
res.statusCode = 400; return ;
}
List<Account> accList = [SELECT Id, Name FROM Account WHERE Id =: SfId];
if(accList != null && !accList.isEmpty()){
res.responseBody = Blob.valueOf(JSON.serialize(new SuccessResponse(accList.get(0).Name, accList.get(0).Id)));
res.statusCode = 200;
} else {
res.responseBody = Blob.valueOf(JSON.serialize(new errorMessageWrapperClass(‘Not Found:’, ‘NOT Found’)));
res.statusCode = 404;
}
} catch(Exception e) {
res.responseBody = Blob.valueOf(JSON.serialize(new errorMessageWrapperClass(‘Unknown Error: ‘, e.getMessage())));
res.statusCode = 500;
}
}
//Wrapper class for Error response
global class errorMessageWrapperClass {
public String errorType {get;set;}
public String errorMessage {get;set;}
public errorMessageWrapperClass (String errorType, String errorMessage){
this.errorType = errorType ;
this.errorMessage = errorMessage ;
}
}
//Wrapper class for response
global class SuccessResponse {
public String Name {get;set;}
public String SfId {get;set;}
public SuccessResponse (String Name, String SfId){
this.Name = Name ;
this.SfId = SfId ;
}
}
}
Create a connected app (New Salesforce org)
Connected apps are a framework that enables the external app to integrate with Salesforce via APIs. So we set up a connected app in New Salesforce Org.
Please use the following configuration to set up a connect app.
After saving, It will provide “Consumer Key” and “Consumer Secret”. We need these for authentication from the old Salesforce org.
Create Authorization Provider (New Salesforce org)
Before creating Named Credentials, We need to set up an Auth Provider in New Salesforce org. Follow the following steps for creating an Auth Provider.
- Navigate to Setup -> Auth. Providers.
- Click on the new Button.
- Select “Salesforce” as Provider Type
- Provide a Name for Auth. Provider.
- Provide “Consumer Key” and “Consumer Secret” from the previous step.
- In “Default Scope” enter the value as “refresh_token full”.
- then Save
NOTE: Update Authorize Endpoint URL with https://test.salesforce.com/services/oauth2/authorize if you want to connect with a sandbox or https://login.salesforce.com/services/oauth2/authorize if your want to connect with a production/Developer org.
After successful save, it will a some Salesforce Configurations.
We need the callback URL from here. We need to put this Callback URL in the Connected App. We have previously created it in the new sandbox.
- Navigate to Setup -> Connected app.
- Edit the Connected App.
- Replace the callback URL with the new callback URL.
Create a Named Credentials (New Salesforce org)
Now we have all the things to set up Named credentials in New Salesforce org. Use the following steps to create Named credentials.
- Navigate to Setup -> Named Credentials.
- Click on the new Button.
- Provide a label.
- In URL, Provide the Salesforce instance URL of New Salesforce org (Or your org url)
- Select “Named Principal” in Identity Type.
- Select “OAuth 2.0” in Authentication Protocol.
- Select the Auth Provider we have created in the previous step in Authentication Provider.
- In scope, enter the value as “refresh_token full”
- Check “Allow Merge Fields in HTTP Body” checkbox.(this is important to add request body to API callout)
- Finally, Save.
After clicking the save button, It will redirect to the Salesforce login page to authenticate the Named Credentials. You need to provide the New Salesforce API User. After successful authentication, you will redirect to Named Credentials and you will see “Authenticated as” in Authentication Status.
If you are facing this issue error=redirect_uri_mismatch&error_description=redirect_uri%20must%20match%20configuration while authenticating Named Credentials. You need to update the Callback URL in the connected app with the auth provider Salesforce configuration.
Apex Code to Connect with New Salesforce using Named Credentials
Now we are ready to use named credentials in Apex class. Following are the apex code example.
HttpRequest req = new HttpRequest();
req.setEndpoint('callout:Your_Named_Credentials/services/apexrest/SampleRestAPI/');
req.setHeader('Content-Type', 'application/json');
req.setMethod('POST');
req.setBody('{"SfId": "' + Your_SfId+ '"}');
Http http = new Http();
HTTPResponse resp = http.send(req);
We hope this article was helpful for you to integrate one org with another.
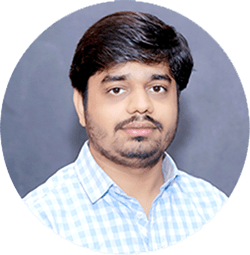
Akhil Mandia
Salesforce Developer
Akhil, one of our sophisticated developers, holds a deep passion for coding and software development. He has a background in website development and has continued to grow his passion in the Salesforce Ohana as a Salesforce Developer.